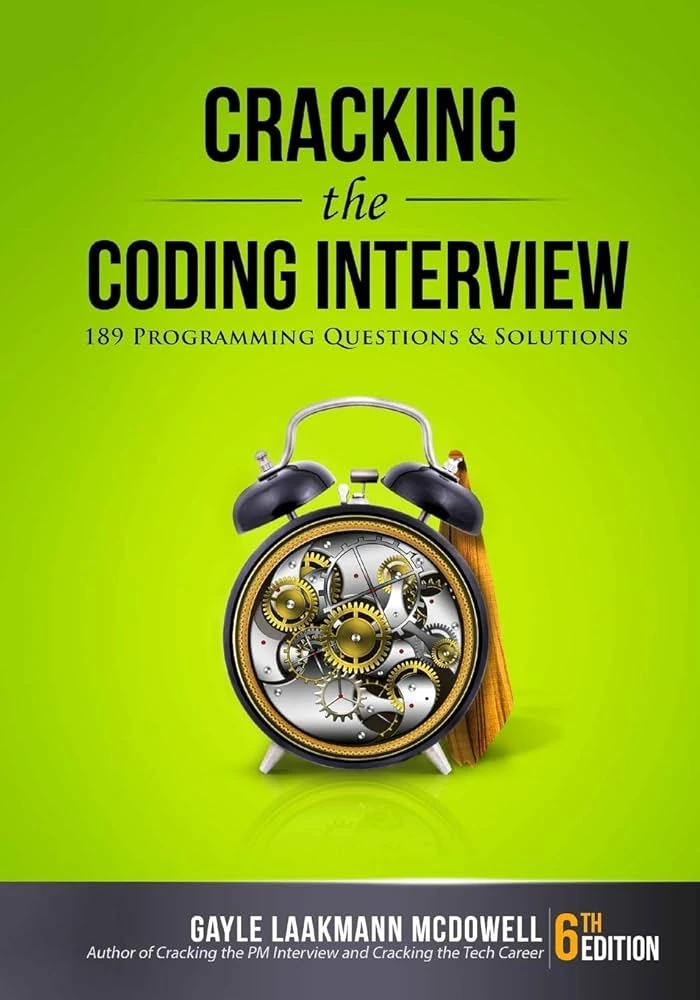
Cracking the Coding Interview
Gayle Laakmann McDowell
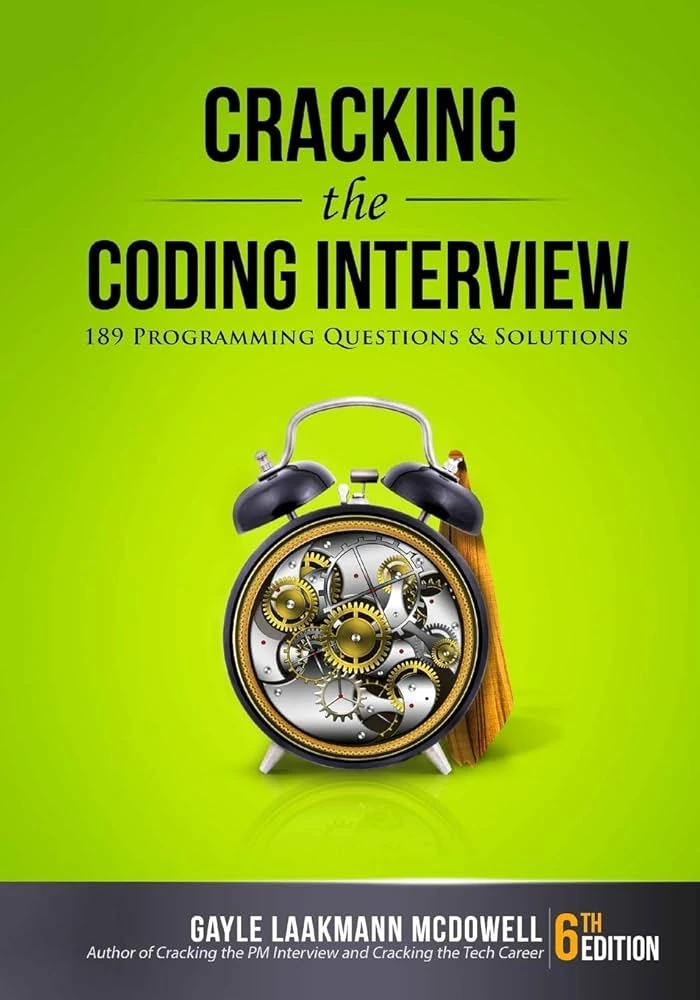
I am not a recruiter. I am a software engineer. And as such, I know what it's like to be asked to whip up brilliant algorithms on the spot and then write flawless code on a whiteboard. I've been through this as a candidate and as an interviewer.
Cracking the Coding Interview, 6th Edition is here to help you through this process, teaching you what you need to know and enabling you to perform at your very best. I've coached and interviewed hundreds of software engineers. The result is this book.
Learn how to uncover the hints and hidden details in a question, discover how to break down a problem into manageable chunks, develop techniques to unstick yourself when stuck, learn (or re-learn) core computer science concepts, and practice on 189 interview questions and solutions.
These interview questions are real; they are not pulled out of computer science textbooks. They reflect what's truly being asked at the top companies, so that you can be as prepared as possible.
LeetCode Problems
Cracking the Coding Interview
Help us improve! If you notice any incorrectly assigned exercises or if you know of any that are currently unassigned, please report it or submit a pull request on GitHub.
Number | Question | Leetcode Number | Leetcode Title | Similar Questions | Difficulty | Video | |
---|---|---|---|---|---|---|---|
Arrays and Strings | |||||||
1.1 | Is Unique | - | - | 217 | N/A | N/A | |
1.2 | Check Permutation | 567 | Permutation in String | 242 | Medium | ||
1.3 | URLify | 1410 | HTML Entity Parser | - | Medium | N/A | |
1.4 | Palindrome Permutation | 266 | Palindrome Permutation | - | Easy | N/A | |
1.5 | One Away | 161 | One Edit Distance | 72 | Medium | N/A | |
1.6 | String Compression | 443 | String Compression | - | Medium | N/A | |
1.7 | Rotate Matrix | 48 | Rotate Image | - | Medium | ||
1.8 | Zero Matrix | 73 | Set Matrix Zeroes | - | Medium | ||
1.9 | String Rotation | 796 | Rotate String | - | Easy | ||
Linked Lists | |||||||
2.1 | Remove Dups | 1836 | Remove Duplicates from an Unsorted Linked List | - | Medium | N/A | |
2.2 | Return Kth to Last | - | - | 19 | N/A | N/A | |
2.3 | Delete Middle Node | 876 | Middle of the Linked List | 237 | Easy | ||
2.4 | Partition | 86 | Partition List | - | Medium | ||
2.5 | Sum Lists | 2 | Add Two Numbers | 445 | Medium | ||
2.6 | Palindrome | 234 | Palindrome Linked List | - | Easy | ||
2.7 | Intersection | 160 | Intersection of Two Linked Lists | - | Easy | ||
2.8 | Loop Detection | 142 | Linked List Cycle II | - | Medium | N/A | |
Stacks and Queues | |||||||
3.1 | Three In One | - | - | - | N/A | N/A | |
3.2 | Stack Min | 155 | Min Stack | - | Medium | ||
3.3 | Stack of Plates | 1172 | Dinner Plate Stacks | - | Hard | N/A | |
3.4 | Queue via Stacks | 232 | Implement Queue using Stacks | - | Easy | ||
3.5 | Sort Stacks | - | - | - | N/A | N/A | |
3.6 | Animal Shelter | - | - | - | N/A | N/A | |
Trees and Graphs | |||||||
4.1 | Route Between Nodes | - | - | 1971 | N/A | N/A | |
4.2 | Minimal Tree | 108 | Convert Sorted Array to Binary Search Tree | - | Easy | ||
4.3 | List of Depths | 102 | Binary Tree Level Order Traversal | - | Medium | ||
4.4 | Check Balanced | 110 | Balanced Binary Tree | - | Easy | ||
4.5 | Validate BST | 98 | Validate Binary Search Tree | - | Medium | ||
4.6 | Successor | 510 | Inorder Successor in BST II | - | Medium | N/A | |
4.7 | Build Order | 210 | Course Schedule II | - | Medium | ||
4.8 | First Common Ancestor | 236 | Lowest Common Ancestor of a Binary Tree | 1644 | Medium | ||
4.9 | BST Sequences | - | - | 1569 | N/A | N/A | |
4.1 | Check Subtree | 572 | Subtree of Another Tree | - | Easy | ||
4.11 | Random Node | - | - | - | N/A | N/A | |
4.12 | Paths with Sum | 437 | Path Sum III | - | Medium | N/A | |
Bit Manipulation | |||||||
5.1 | N/A | - | - | - | N/A | N/A | |
5.2 | N/A | - | - | - | N/A | N/A | |
5.3 | N/A | - | - | - | N/A | N/A | |
5.4 | N/A | - | - | - | N/A | N/A | |
5.5 | N/A | - | - | - | N/A | N/A | |
5.6 | N/A | - | - | - | N/A | N/A | |
5.7 | N/A | - | - | - | N/A | N/A | |
5.8 | N/A | - | - | - | N/A | N/A | |
Recursion and Dynamic Programming | |||||||
8.1 | Triple Step | 1137 | N-th Tribonacci Number | - | Easy | ||
8.2 | Robot in a Grid | 63 | Unique Paths II | - | Medium | ||
8.3 | Magic Index | - | - | - | N/A | N/A | |
8.4 | Power Set | 78 | Subsets | - | Medium | ||
8.5 | Recursive Multiply | - | - | 29 | N/A | N/A | |
8.6 | Towers of Hanoi | - | - | - | N/A | N/A | |
8.7 | Permutation without Dups | 46 | Permutations | - | Medium | ||
8.8 | Permutation with Duplicates | 47 | Permutations II | - | Medium | ||
8.9 | Parens | 22 | Generate Parentheses | - | Medium | ||
8.1 | Paint Fill | 733 | Flood Fill | - | Easy | ||
8.11 | Coins | 518 | Coin Change 2 | - | Medium | ||
8.12 | Eight Queens | 51 | N-Queens | - | Hard | ||
8.13 | Stack of Boxes | - | - | 1691 | N/A | N/A | |
8.14 | Boolean Evaluation | - | - | - | N/A | N/A | |
Sorting and Searching | |||||||
10.1 | Sorted Merge | 88 | Merge Sorted Array | - | Easy | ||
10.2 | Group Anagrams | 49 | Group Anagrams | - | Medium | ||
10.3 | Search in Rotated Array | 81 | Search in Rotated Sorted Array II | 33 | Medium | ||
10.4 | Sorted Search | 702 | Search in a Sorted Array of Unknown Size | - | Medium | N/A | |
10.5 | Sparse Search | - | - | - | N/A | N/A | |
10.6 | Sort Big File | - | - | - | N/A | N/A | |
10.7 | Missing Int | - | - | 268 | N/A | N/A | |
10.8 | Find Duplicates | 287 | Find the Duplicate Number | - | Medium | ||
10.9 | Sorted Matrix Search | 240 | Search a 2D Matrix II | - | Medium | N/A | |
10.1 | Rank from Stream | - | - | 703 | N/A | N/A | |
10.11 | Peaks and Valleys | 280 | Wiggle Sort | - | Medium | ||
Moderate | |||||||
16.1 | Number Swapper | - | - | - | N/A | N/A | |
16.2 | Word Frequencies | 192 | Word Frequency | - | Medium | N/A | |
16.3 | Intersection | - | - | - | N/A | N/A | |
16.4 | Tic Tac Win | 1275 | Find Winner on a Tic Tac Toe Game | - | Easy | N/A | |
16.5 | Factorial Zeros | 172 | Factorial Trailing Zeroes | - | Medium | N/A | |
16.6 | Smallest Difference | - | - | - | N/A | N/A | |
16.7 | Number Max | - | - | - | N/A | N/A | |
16.8 | English Int | 273 | Integer to English Words | - | Hard | ||
16.9 | Operations | - | - | - | N/A | N/A | |
16.1 | Living People | - | - | - | N/A | N/A | |
16.11 | Diving Board | - | - | - | N/A | N/A | |
16.12 | XML Encoding | - | - | - | N/A | N/A | |
16.13 | Bisect Squares | - | - | - | N/A | N/A | |
16.14 | Best Line | 149 | Max Points on a Line | - | Hard | ||
16.15 | Master Mind | - | - | - | N/A | N/A | |
16.16 | Sub Sort | 581 | Shortest Unsorted Continuous Subarray | - | Medium | N/A | |
16.17 | Contiguous Sequence | 53 | Maximum Subarray | - | Medium | ||
16.18 | Pattern Matcher | - | - | 290 | N/A | N/A | |
16.19 | Pond Sizes | - | - | 200 | N/A | N/A | |
16.2 | T9 | - | - | 17 | N/A | N/A | |
16.21 | Sum Swap | - | - | - | N/A | N/A | |
16.22 | Langtons Ant | - | - | - | N/A | N/A | |
16.23 | Rand7 From Rand5 | 470 | Implement Rand10() Using Rand7() | - | Medium | N/A | |
16.24 | Pairs With Sum | 1679 | Max Number of K-Sum Pairs | - | Medium | N/A | |
16.25 | LRU Cache | 146 | LRU Cache | - | Medium | ||
16.26 | Calculator | 227 | Basic Calculator II | - | Medium | N/A | |
Hard | |||||||
17.1 | Add Without Plus | 371 | Sum of Two Integers | N/A | Medium | ||
17.2 | Shuffle | 384 | Shuffle an Array | - | Medium | N/A | |
17.3 | Random Set | - | - | - | N/A | N/A | |
17.4 | Missing Number | - | - | 268 | N/A | N/A | |
17.5 | Letters and Numbers | 525 | Contiguous Array | - | Medium | ||
17.6 | Count of 2s | - | - | - | N/A | N/A | |
17.7 | Baby Names | - | - | - | N/A | N/A | |
17.8 | Circus Tower | - | - | - | N/A | N/A | |
17.9 | Kth Multiple | - | - | - | N/A | N/A | |
17.1 | Majority Element | 169 | Majority Element | - | Easy | ||
17.11 | Word Distance | 244 | Shortest Word Distance II | 243 | Medium | N/A | |
17.12 | BiNode | 426 | Convert Binary Search Tree to Sorted Doubly Linked List | - | Medium | N/A | |
17.13 | ReSpace | 140 | Word Break II | 139 | Hard | ||
17.14 | Smallest K | 215 | Kth Largest Element in an Array | - | Medium | ||
17.15 | Longest Word | - | - | 472 | N/A | N/A | |
17.16 | The Masseuse | 198 | House Robber | - | Medium | ||
17.17 | Multi Search | - | - | - | N/A | N/A | |
17.18 | Shortest Supersequence | - | - | 727 | N/A | N/A | |
17.19 | Missing Two | - | - | 268 | N/A | N/A | |
17.2 | Continuous Median | 295 | Find Median from Data Stream | - | Hard | ||
17.21 | Volume of Histogram | 42 | Trapping Rain Water | - | Hard | ||
17.22 | Word Transformer | 127 | Word Ladder | - | Hard | ||
17.23 | Max Black Square | 221 | Maximal Square | - | Medium | ||
17.24 | Max Submatrix | - | - | - | N/A | N/A | |
17.25 | Word Rectangle | - | - | - | N/A | N/A | |
17.26 | Sparse Similarity | - | - | - | N/A | N/A |